Best Practices for Optimized ReactJS Development

Master Component Nesting and the Parent-Child Relationships
2.Optimizing Re-Renders
When Using PureComponent
Using React.memo
3.Prop Drilling and Context API
4.Eliminate The Code Duplication
const buttons = ['facebook', 'twitter', 'youtube'];
return (
{
buttons.map( (button) => {
return (
);
} )
}
);
5.Improving Code Reusability with Naming Conventions
import React from 'react';
function StudentList() {
return (
StudentList
)
}
export default StudentList
6.Use Linter Wisely with Code Quality Enhancement
The Linter tool is used by most developers, which enhances the quality of the code. ESLint is among the most widely used Linter tools for React. It may benefit the code base’s consistency. Additionally, you can specify in developer rules that double quotations rather than single quotes are required.
It might also have naming conventions, brackets around arrow functions, etc. The program watches the code and alerts users when a rule is broken. Additionally, the rule-breaking keyword is highlighted in red. Code homogeneity can be improved with the use of linter tools. As you code, it can assist in automatically fixing errors.
7.Utilize Fragments with Lesser Load and More Performance
Tips To Maintain Code and Functionality For React Developers
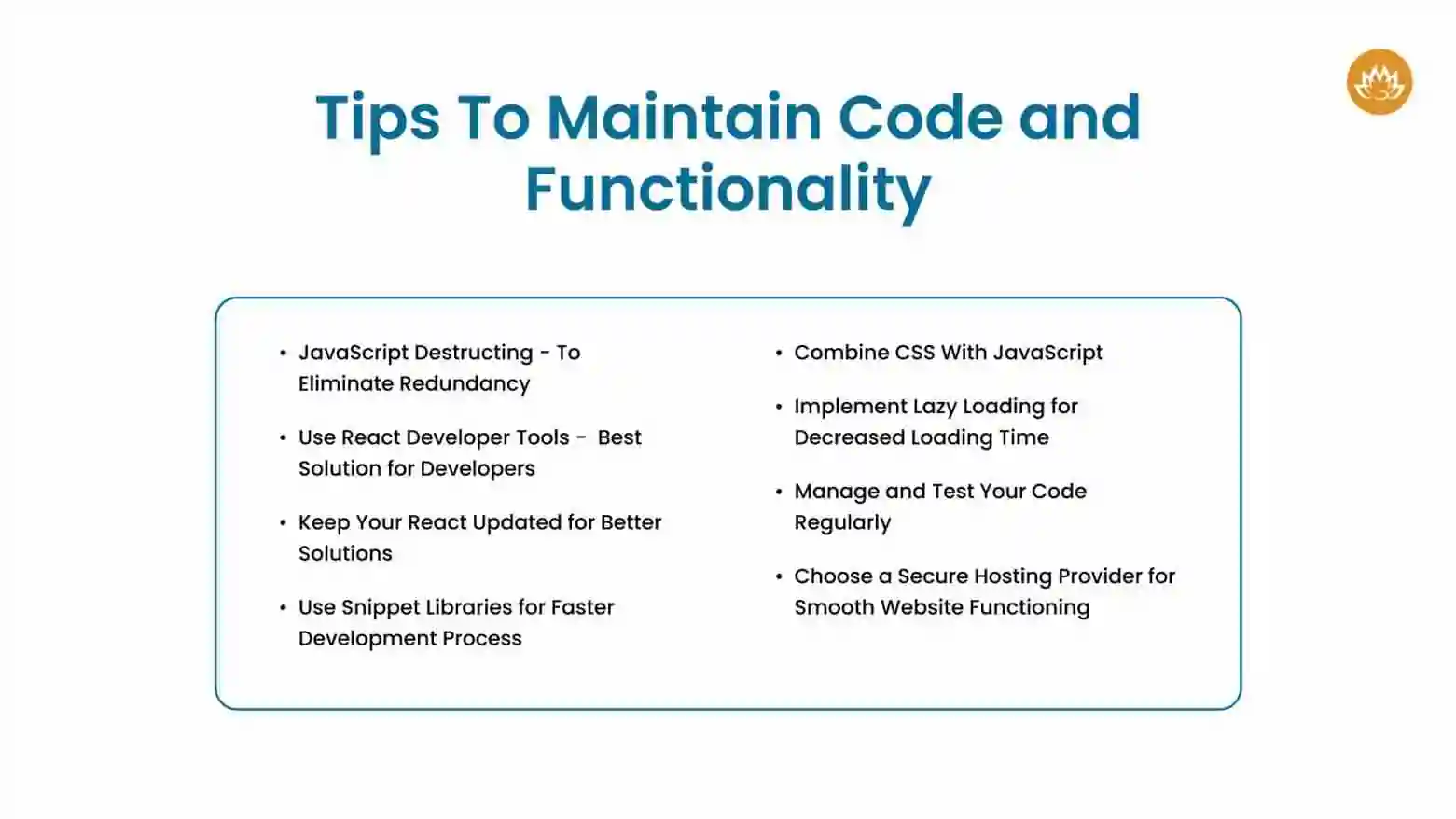
1.JavaScript Destructing - To Eliminate Redundancy
2.Use React Developer Tools - Best Solution for Developers
3.Keep Your React Updated for Better Solutions
4.Use Snippet Libraries for Faster Development Process
5.Combine CSS With JavaScript
resource edge
6.Implement Lazy Loading for Decreased Loading Time
For example, you might load the modules as the necessary bits for registration and login, and the rest might load according to user navigation. Additionally, certain developers use lazy loading for photos and videos, which speeds up the loading process.
7.Manage and Test Your Code Regularly
8.Choose a Secure Hosting Provider for Smooth Website Functioning
Conclusion
Author
Sunil is a result-orientated Chief Technology Officer with over a decade of deep technical experience delivering solutions to startups, entrepreneurs, and enterprises across the globe. Have led large-scale projects in mobile and web applications using technologies such as React Native, Flutter, Laravel, MEAN and MERN stack development.
View all posts